Visitor Design Pattern
Structural: Visitor Pattern
What is the Visitor Design Pattern in JavaScript?
View Answer:
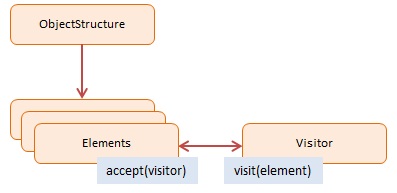
Below is an example of the Visitor design pattern in JavaScript:
class ObjectStructure {
constructor() {
this.nodes = [];
}
add(node) {
this.nodes.push(node);
}
accept(visitor) {
this.nodes.forEach(node => node.accept(visitor));
}
}
class NodeA {
accept(visitor) {
visitor.visitNodeA(this);
}
operationA() {
return 'NodeA is visited';
}
}
class NodeB {
accept(visitor) {
visitor.visitNodeB(this);
}
operationB() {
return 'NodeB is visited';
}
}
class Visitor {
visitNodeA(node) {
console.log(node.operationA());
}
visitNodeB(node) {
console.log(node.operationB());
}
}
// Usage
const objectStructure = new ObjectStructure();
objectStructure.add(new NodeA());
objectStructure.add(new NodeB());
const visitor = new Visitor();
// This will run the corresponding visitor method for each node in the object structure
objectStructure.accept(visitor);
In this example, we have a couple of node classes (NodeA
and NodeB
) each with a method accept
that accepts a visitor object. The visitor has a method for each type of node that it can visit (visitNodeA
and visitNodeB
). These methods are then called on each of the nodes in the ObjectStructure
when the accept
method is called on the ObjectStructure
, passing the visitor as a parameter. The result is that the corresponding method on the visitor is called for each type of node in the ObjectStructure
.
This is a simple example, and the real value of the Visitor pattern comes when the object structure and/or the algorithms being separated out are more complex, but this should give you a basic idea of how it works.
To which pattern family does the Visitor pattern belong?
View Answer:
Why use the Visitor Design Pattern?
View Answer:
We can use the visitor pattern when:
- Similar procedures must get done on various data structure objects.
- Specific operations must get carried out on multiple items in the data structure.
- You wish to make libraries or frameworks more extensible.
What are some of the benefits of using the Visitor pattern?
View Answer:
- The principle of open/closed. You may add new behavior that works with objects of various classes without modifying the classes themselves.
- Single Responsibility Principle. You can move multiple versions of the same behavior into the same class.
- While working with various objects, a visitor object might get helpful information. This information is helpful if you wish to traverse a complicated object structure, such as an object tree, and apply the Visitor to each item in the structure.
What are some of the Visitor pattern's drawbacks?
View Answer:
- Every time a class is added or withdrawn from the element hierarchy, you must notify all visitors.
- Visitors may not have access to the private fields and methods of the components they get expected to operate.
Are there any alternatives to using the Visitor pattern?
View Answer:
What problems does the Visitor Pattern solve?
View Answer:
How does the Visitor Pattern achieve separation of concerns?
View Answer:
When might the Visitor Pattern not be ideal?
View Answer:
Can you name a real-world scenario where the Visitor Pattern would be beneficial?
View Answer:
How does the Visitor Pattern help in extending functionality?
View Answer:
What are the main components of the Visitor Design Pattern?
View Answer:
Visitor
: This is an interface or abstract class that declares avisit
method for each type ofVisitable
object.ConcreteVisitor
: This is a concrete class that implements theVisitor
interface or extends the abstractVisitor
class. It implements thevisit
method for each type ofVisitable
object.Visitable
orElement
: This is an interface or abstract class that declares theaccept
method, which takes aVisitor
as an argument.ConcreteElement
: This is a concrete class that implements theVisitable
orElement
interface or extends the abstractElement
class. It implements theaccept
method.ObjectStructure
: This is a collection ofConcreteElements
. It can enumerate its elements and may provide a high-level interface to allow the visitor to visit its elements.
Code Example:
// Visitable or Element
class Employee {
constructor(name, salary) {
this.name = name;
this.salary = salary;
}
accept(visitor) {
visitor.visit(this);
}
}
// ConcreteElement
class Developer extends Employee {
constructor(name, salary) {
super(name, salary);
}
}
// ConcreteElement
class Manager extends Employee {
constructor(name, salary) {
super(name, salary);
}
}
// Visitor
class Payroll {
visit(employee) {
let monthlySalary;
if (employee instanceof Manager) {
monthlySalary = employee.salary / 12;
console.log(`${employee.name}'s monthly salary is ${monthlySalary.toFixed(2)}`);
}
if (employee instanceof Developer) {
monthlySalary = employee.salary / 12;
console.log(`${employee.name}'s monthly salary is ${monthlySalary.toFixed(2)}`);
}
}
}
// ObjectStructure
class Employees {
constructor() {
this.employees = [];
}
addEmployee(employee) {
this.employees.push(employee);
}
accept(visitor) {
this.employees.forEach(employee => employee.accept(visitor));
}
}
// Usage
let employees = new Employees();
employees.addEmployee(new Manager('John Doe', 120000));
employees.addEmployee(new Developer('Jane Doe', 80000));
let payroll = new Payroll();
employees.accept(payroll);
In this example, Employee
is the Visitable
or Element
class, Developer
and Manager
are the ConcreteElement
classes, Payroll
is the Visitor
or ConcreteVisitor
, and Employees
is the ObjectStructure
. The accept
method in the Employee
class lets a Visitor
object visit it, in this case, to calculate monthly salaries. The Payroll
class implements the visit
method to calculate and print the monthly salary for each type of Employee
. The Employees
class represents an object structure that can enumerate its elements and allow a visitor to visit them.