Interpreter Design Pattern
Structural: Interpreter Pattern
What is the Interpreter design pattern in JavaScript?
View Answer:
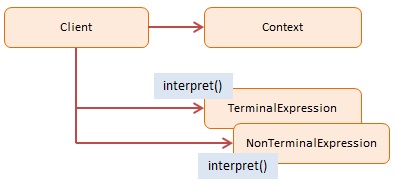
The objects participating in this pattern are:
Client -- Example code: the run() program.
- creates (or is provided) a syntax tree that represents the grammar
- establishes the starting point context for the interpreter
- makes use of the interpret operations
Context -- Example code: Context
- It provides the interpreter with state information
TerminalExpression -- Example code: Expression
- performs an interpret operation in conjunction with grammar terminal symbols
- one instance for each phrase's terminal expression
NonTerminalExpression -- In example code: not used
- implements an interpret operation in the grammar for non-terminal symbols
Here is an example of how we can implement the Interpreter pattern in JavaScript to interpret Roman numerals.
In this example, we will have classes to interpret each Roman numeral (I, V, X, L, C, D, M). Then, we use these interpreters to translate a Roman numeral string to an integer.
class Interpreter {
constructor() {
this.grammar = {
"M": 1000,
"D": 500,
"C": 100,
"L": 50,
"X": 10,
"V": 5,
"I": 1
};
}
interpret(input) {
let output = 0;
for(let i = 0; i < input.length; i++) {
let current = this.grammar[input[i]];
let next = this.grammar[input[i + 1]];
if(next && current < next) {
output -= current;
} else {
output += current;
}
}
return output;
}
}
// Usage
let interpreter = new Interpreter();
console.log(interpreter.interpret('MCMXCIV')); // Output: 1994
console.log(interpreter.interpret('IX')); // Output: 9
console.log(interpreter.interpret('LVIII')); // Output: 58
In this example, the interpret
function walks through the input string, adding the current symbol value to the total output, or subtracting if the next symbol's value is larger. By doing so, it's able to correctly interpret Roman numeral strings.
What's the basic structure of the Interpreter pattern?
View Answer:
In which scenarios can we use the Interpreter pattern?
View Answer:
In what pattern category does the Interpreter pattern belong?
View Answer:
What are some of the advantages of employing the Interpreter pattern?
View Answer:
Technical Response: Benefits of the Interpreter Pattern.
Tested and used solution
- It's a tried-and-true, reusable solution used in several different applications.
- Because the pattern uses classes to describe grammatical rules, updating and extending the grammar is simple.
Extendable and straightforward to modify
- Because the pattern describes grammatical rules using classes, updating and extending the grammar is trivial. Using inheritance, you can edit or expand the grammar.
- Existing expressions can be changed progressively: we define new expressions as variants of existing ones.
Simple to implement
- Putting the grammar into practice is similarly straightforward. Classes that define nodes in the abstract syntax tree have comparable implementations.
- These classes are simple to write, and they are typically generated automatically by a compiler or parser generator.
What are some of the disadvantages of employing the Interpreter pattern?
View Answer:
- Because grammar with many rules can be challenging to manage and maintain, the Interpreter pattern creates at least one class for each rule in the grammar.
- Other design patterns can be used to mitigate the problem, however when the language is complex, other approaches such as parser or compiler generators are more suited.
Are there any alternatives to using the Interpreter Pattern?
View Answer:
How does the Interpreter pattern relate to the Composite pattern?
View Answer:
What are Terminal and NonTerminal expressions in the Interpreter pattern?
View Answer:
Here is an example of an arithmetic expression interpreter using modern JavaScript.
class TerminalExpression {
constructor(value) {
this.value = value;
}
interpret() {
return this.value;
}
}
class AddExpression {
constructor(expr1, expr2) {
this.expr1 = expr1;
this.expr2 = expr2;
}
interpret() {
return this.expr1.interpret() + this.expr2.interpret();
}
}
class MultiplyExpression {
constructor(expr1, expr2) {
this.expr1 = expr1;
this.expr2 = expr2;
}
interpret() {
return this.expr1.interpret() * this.expr2.interpret();
}
}
// Terminal expressions
let five = new TerminalExpression(5);
let three = new TerminalExpression(3);
// Non-terminal expressions
let add = new AddExpression(five, three);
let multiply = new MultiplyExpression(add, three);
console.log(multiply.interpret()); // Output: 24
In this example, TerminalExpression
represents terminal expressions, which are the numbers themselves. AddExpression
and MultiplyExpression
are non-terminal expressions, they represent arithmetic operations that involve other expressions.