Object to Primitive Conversion
Objects the Basics: Object to Primitive Conversion
What is Object to Primitive Conversion in JavaScript?
View Answer:
Interview Response: Object to Primitive Conversion in JavaScript is the process of converting an object to a primitive value (string, number, or boolean) when it is used in a context that expects a primitive.
Code Example:
const obj = {
valueOf() {
return 42;
},
toString() {
return "Object Value";
}
};
console.log(obj + 10); // Output: 52
console.log(String(obj)); // Output: "Object Value"
What is the rule for objects in a Boolean context?
View Answer:
Interview Response: In a boolean context, all objects are considered truthy. When coerced to a boolean, they evaluate to true, regardless of their properties or content.
Technical Response: In JavaScript, the general rule is that when an object is converted to a boolean context (for instance, in a conditional like an `if` statement), it is considered `true`, regardless of its content. This includes empty objects `` and empty arrays `[]`.
Code Example:
let obj = {};
if (obj) {
console.log("The object is truthy!");
} else {
console.log("The object is falsy!");
}
What are the three variants of type conversion in JavaScript?
View Answer:
Interview Response: String, Number, and Boolean conversions are the three variants of primitive type conversion in JavaScript.
Technical Response: The three variants of type conversion include string, number, and default conversions. String conversion can happen explicitly when an object expects a string, and mathematical operations use explicit number conversion on primitives. In rare circumstances where the operator is unclear about what type to anticipate, the default gets used.
JavaScript has three type conversion methods:
String Conversion:
let value = true;
console.log(typeof value); // boolean
value = String(value); // now value is a string "true"
console.log(typeof value); // string
Number Conversion:
let str = "123";
console.log(typeof str); // string
let num = Number(str); // becomes a number 123
console.log(typeof num); // number
Boolean Conversion:
console.log(Boolean(1)); // true
console.log(Boolean(0)); // false
console.log(Boolean("hello")); // true
console.log(Boolean("")); // false
All these conversions are mostly plain and intuitive except for some peculiar cases like null
and undefined
, which are non-numeric, non-string (except when null and undefined are implicitly converted to string), and falsy.
What are the three variants of Object to Primitive Conversion in JavaScript?
View Answer:
Interview Response: In JavaScript, Object to Primitive Conversion has three variants: ToPrimitive for general conversion, which calls valueOf or toString methods; ToNumber for numeric conversion, which also calls valueOf or toString methods; and ToString for string conversion, which calls toString or valueOf methods.
Technical Details: The process by which JavaScript tries to convert an object to a primitive value is called "object-to-primitive conversion". It's used when an object is used in a context where a primitive value is required, like in alert, mathematical operations, comparisons, etc.
Code Example:
let user = {
name: "John",
money: 1000,
[Symbol.toPrimitive](hint) {
console.log(`hint: ${hint}`);
return hint == "string" ? `{name: "${this.name}"}` : this.money;
}
};
// conversions demo:
console.log(user); // hint: string -> {name: "John"}
console.log(+user); // hint: number -> 1000
console.log(user + 500); // hint: default -> 1500
What methods are called and in what order during Object to Primitive Conversion?
View Answer:
Interview Response: During Object to Primitive Conversion, JavaScript tries to call the methods valueOf(), toString(), and Symbol.toPrimitive() in that order.
ToPrimitive Algorithm:
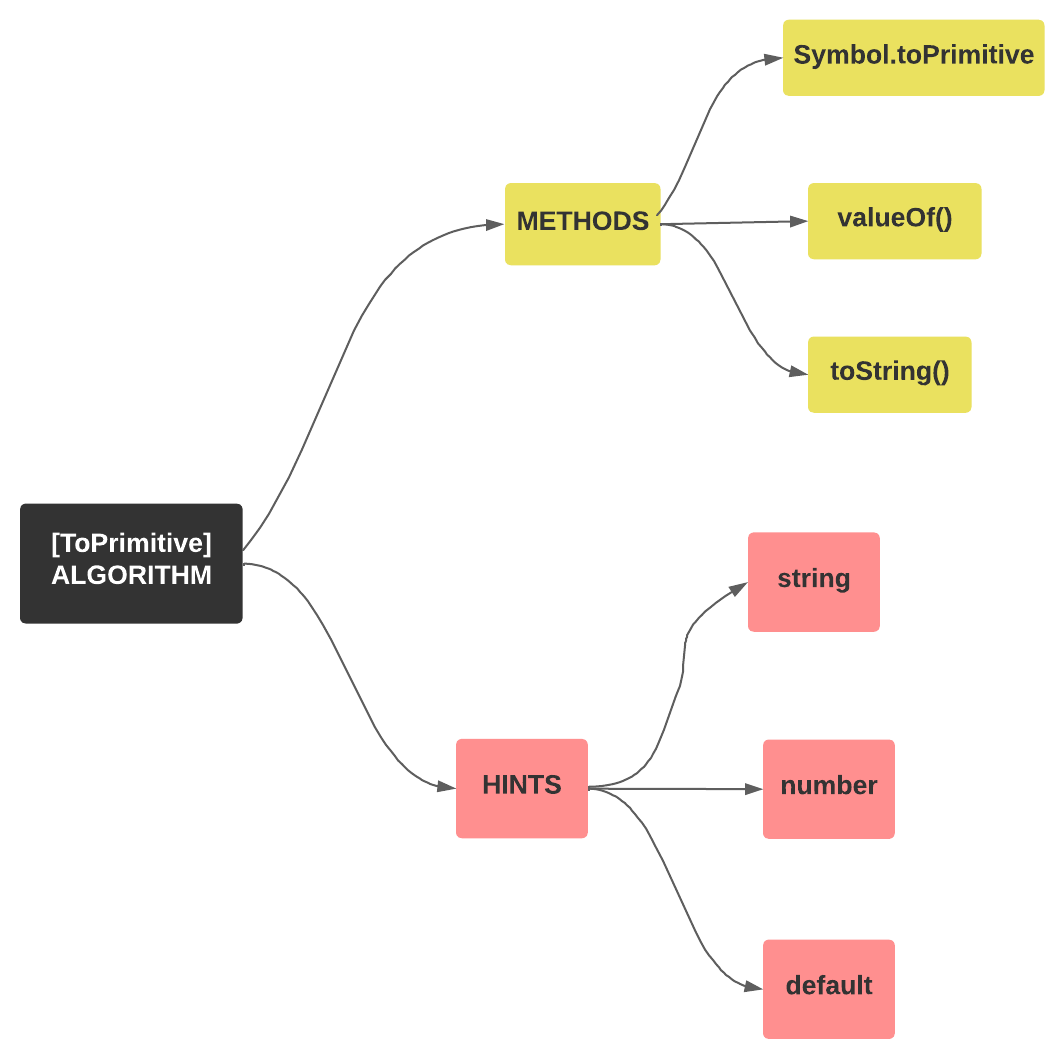
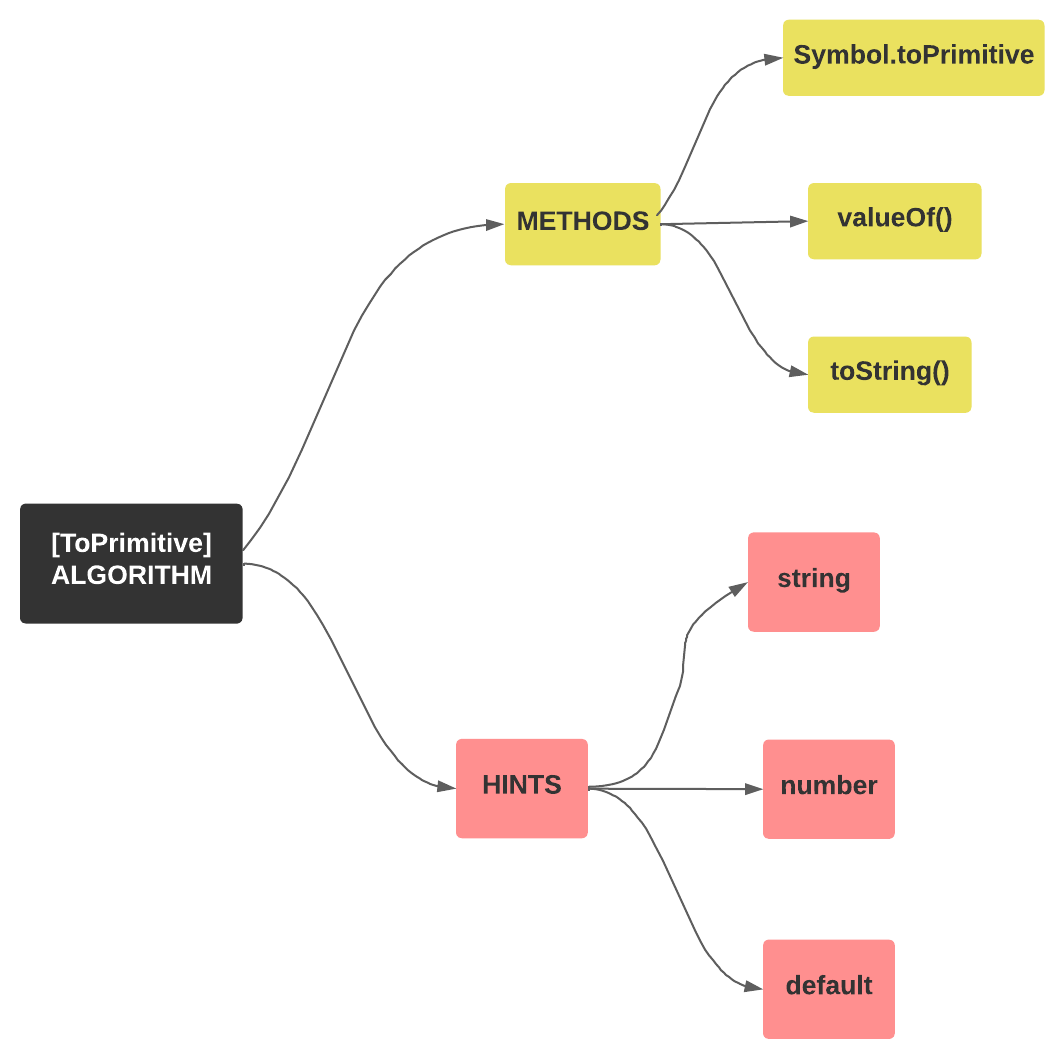
To implement conversions, what are the three object methods JavaScript tries to find and call?
View Answer:
Interview Response: The three object methods include Symbol.toPrimtive (system symbol) if it exists. Otherwise, if the hint is a string, it will try Obj.toString() or Obj.valueOf(). Finally, if the hint is a number or default it will try Obj.valueOf() and Obj.toString().
Simplified: The three object methods include Symbol.toPrimitive, Obj.toString(), and/or Obj.valueOf().
Can you explain what Symbol.toPrimitive() is and what it does?
View Answer:
Interview Response: Symbol.toPrimitive() is a method that can be defined on an object to customize its conversion to a primitive value. It is called by the ToPrimitive() abstract operation.
Code Example:
let user = {
name: 'John',
money: 1000,
[Symbol.toPrimitive](hint) {
console.log(`hint: ${hint}`);
return hint == 'string' ? `{name: "${this.name}"}` : this.money;
},
};
// conversions demo:
console.log(user); // hint: string -> {name: "John"}
console.log(+user); // hint: number -> 1000
console.log(user + 500); // hint: default -> 1500
What is the outcome when you try to use a for…loop to expose the properties of an object using Symbol.toPrimitive()?
View Answer:
Interview Response: The result returns all properties except for the Symbol.toPrimitive because JavaScript does not expose Symbols in the global symbol registry in this fashion.
Code Example:
let user = {
name: 'John',
money: 1000,
[Symbol.toPrimitive](hint) {
console.log(`hint: ${hint}`);
return hint == 'string' ? `{name: "${this.name}"}` : this.money;
},
};
for (let prop in user) {
console.log(prop); // returns name, money but no Symbol
}
Both toString and valueOf come from ancient times. Are these Symbols?
View Answer:
Interview Response: No, because toString and valueOf came before Symbols debuted in the JavaScript codebase. They are regular string-name methods.
Technical Response: No, toString and valueOf are not considered Symbols in JavaScript. They are standard methods that come from JavaScript's Object.prototype and have been in the language since its inception.
note
In ECMAScript 2015 (ES6), the Symbol.toPrimitive method was introduced as a way to customize primitive value conversion in a more modern and comprehensive way.
A plain object has both toString and valueOf methods. What do they return?
View Answer:
Interview Response: By default, a plain object's toString method returns "[object Object]", a string representation, and the valueOf method returns the object itself, without any conversion.
Code Example:
let user = { name: 'John' };
console.log(user); // [object Object]
console.log(user.valueOf() === user); // true
What happens if toString or valueOf returns an object?
View Answer:
Interview Response: There is no error, but such value gets ignored.
Technical Response: For historical reasons, if toString or valueOf returns an object, there is no error, but such value is ignored (like if the method did not exist). That is because, in ancient times, there was no good "error" concept in JavaScript.
As you pass an object as an argument, what are the stages?
View Answer:
Interview Response: The object gets converted to a primitive. If the resulting primitive is not the right type, it gets converted.
Technical Response: When passing an object as an argument, these stages occur: argument evaluation, object-to-primitive conversion if required by the function, function execution, and return value processing.
- The object gets converted into a primitive.
- If the resulting primitive is not of the right type, it gets converted.
Code Example:
let obj = {
// toString handles all conversions in the absence of other methods
toString() {
return '2';
},
};
console.log(obj * 2); // 4, object converted to primitive "2", then multiplication made it a number
When passing an object as an argument, how is it passed in JavaScript?
View Answer:
Interview Response: In JavaScript, when passing an object as an argument, it's passed by reference, meaning the function receives a reference to the original object, not a copy. This means that changes to the object will persist outside of the function.