Prototype Design Pattern
Creational: Prototype Pattern
What is the Prototype Design Pattern in JavaScript?
View Answer:
We can use the prototype pattern to create new objects based on its blueprint by cloning an existing object. The prototype pattern based on prototypal inheritance can use JavaScript's native prototyping capabilities.
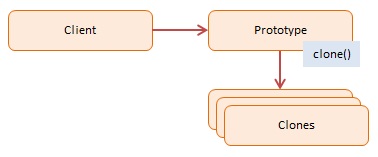
The objects participating in this pattern are:
Client -- In example code: the run() function
- creates a new object by asking a prototype to clone itself
Prototype -- In example code: CustomerPrototype
- creates an interfaces to clone itself
Clones -- In example code: Customer
- the cloned objects that are being created
In JavaScript, every object has a prototype from which it can inherit properties and methods. The Object.create()
method is one way to implement the Prototype Design Pattern.
Here's an example:
var carPrototype = {
start: function () {
return 'Engine of ' + this.model + ' starting...';
},
stop: function () {
return 'Engine of ' + this.model + ' stopping...';
}
};
function Car(model, year) {
this.model = model;
this.year = year;
}
Car.prototype = carPrototype;
var car1 = new Car('Toyota Corolla', 2005);
console.log(car1.start()); // Engine of Toyota Corolla starting...
In this example, carPrototype
is the prototype object with methods common to all cars, start()
and stop()
. The Car
function is a constructor that creates a new car. It sets the prototype of the newly created object to carPrototype
using Car.prototype = carPrototype;
.
By using the prototype, any car we create has access to the start
and stop
methods. This allows us to have common functionality across all instances of a type (in this case, Car
), while still allowing individual instances to have their own properties (in this case, model
and year
).
The Prototype pattern belongs to which design pattern family?
View Answer:
What is an example of a good use case for the prototype pattern?
View Answer:
function Book(title, author, genre, publicationDate) {
this.title = title;
this.author = author;
this.genre = genre;
this.publicationDate = publicationDate;
// Let's assume that getting these details is a complex task, maybe involving a database call or complex computations
this.getDetails = function() {
// complex code goes here...
return this.title + ' by ' + this.author + ', ' + this.genre + ', published on ' + this.publicationDate;
}
}
var book1 = new Book('The Great Book', 'John Doe', 'Science Fiction', '2001-01-01');
If you need to create a new book that shares some properties with book1
, you could create a new Book
object and pass in the required details. However, if the initialization process is complex and resource-intensive, creating a new Book
from scratch may not be the most efficient approach.
In such cases, you can use the Prototype Pattern to clone the existing book and just modify the properties that differ:
function clone(source) {
var Constructor = source.constructor;
var prototype = Object.create(Constructor.prototype);
var clone = new Constructor();
for (var attr in source) {
if (source.hasOwnProperty(attr)) {
clone[attr] = source[attr];
}
}
return clone;
}
var book2 = clone(book1);
book2.title = 'Another Great Book';
book2.author = 'Jane Doe';
console.log(book2.getDetails()); // Another Great Book by Jane Doe, Science Fiction, published on 2001-01-01
In this example, the clone
function creates a new object that is a copy of an existing object and allows you to modify the new object as needed. This approach reduces the cost of initializing a new object when it shares most of its properties and behavior with an existing object.
What are some of the advantages of employing the Prototype pattern?
View Answer:
- We can clone an object without being bound to its concrete classes.
- You can avoid repeating the initialization code by cloning pre-built prototypes.
- It is easier to produce complex objects.
- When dealing with structural presets for complex objects, we produce an alternative to inheritance.